Quick Start
The Basic Request Flow
Request Sequence
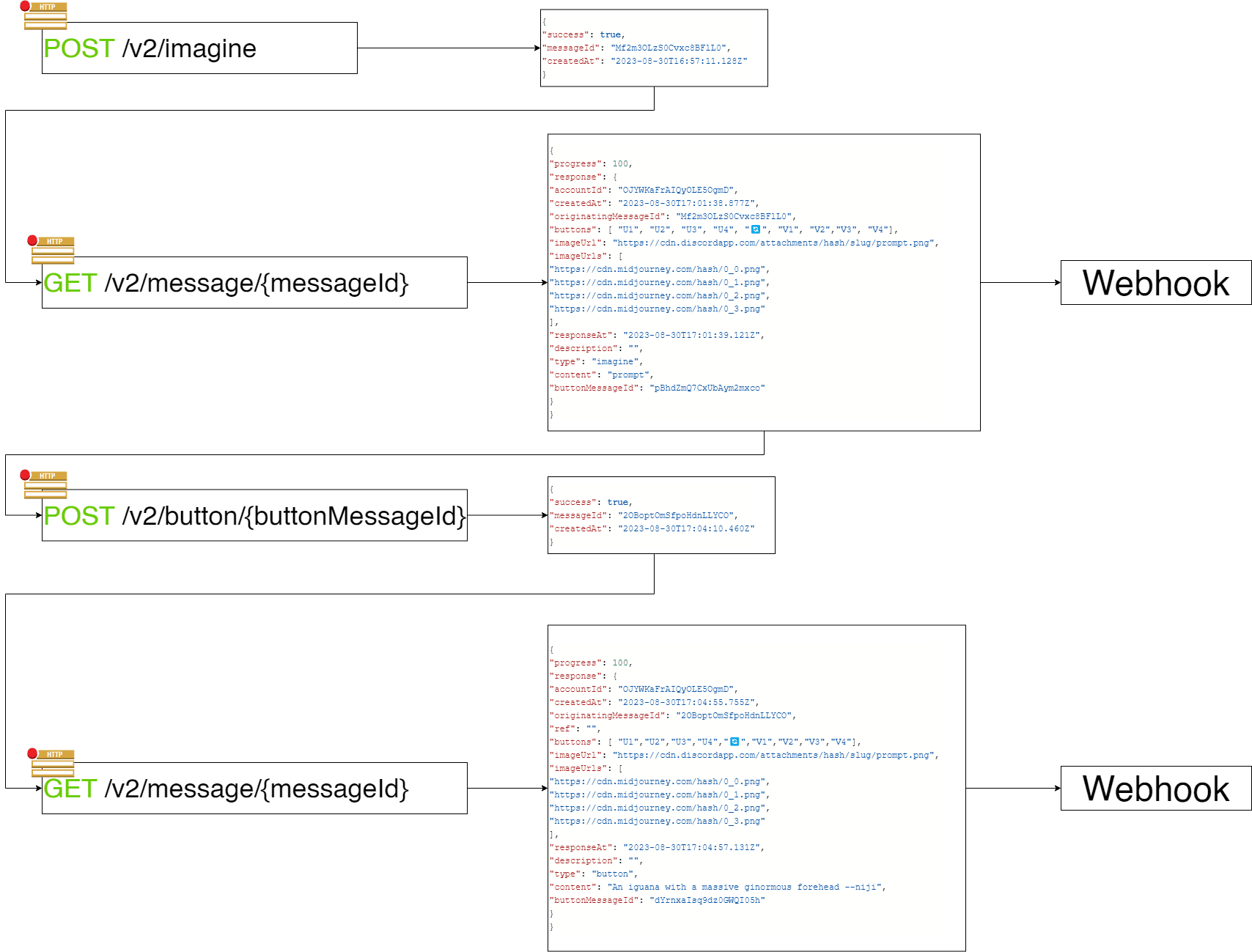
The Code
0
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
const BASE_URL = 'https://api.thenextleg.io/v2';
const AUTH_TOKEN = '';
const AUTH_HEADERS = {
Authorization: `Bearer ${AUTH_TOKEN}`,
'Content-Type': 'application/json',
};
/**
* A function to pause for a given amount of time
*/
function sleep(milliseconds) {
return new Promise(resolve => setTimeout(resolve, milliseconds));
}
/**
* Continue polling a generation an image is completed, or fails.
* You can also use a webhook to get notified when the image is ready.
* It will contain the same response body as seen here.
*/
const fetchToCompletion = async (messageId, retryCount, maxRetry = 20) => {
const imageRes = await fetch(`${BASE_URL}/message/${messageId}`, {
method: 'GET',
headers: AUTH_HEADERS,
});
const imageResponseData = await imageRes.json();
if (imageResponseData.progress === 100) {
return imageResponseData;
}
if (imageResponseData.progress === 'incomplete') {
throw new Error('Image generation failed');
}
if (retryCount > maxRetry) {
throw new Error('Max retries exceeded');
}
if (imageResponseData.progress && imageResponseData.progressImageUrl) {
console.log('---------------------');
console.log(`Progress: ${imageResponseData.progress}%`);
console.log(`Progress Image Url: ${imageResponseData.progressImageUrl}`);
console.log('---------------------');
}
await sleep(5000);
return fetchToCompletion(messageId, retryCount + 1);
};
// we wrap it in a main function here so we can use async/await inside of it.
async function main(
prompt = 'A Rhinoceros in the Amazon with sun shining through, photorealistic, 4k',
) {
/**
* GENERATE THE IMAGE
*/
const imageRes = await fetch(`${BASE_URL}/imagine`, {
method: 'POST',
headers: AUTH_HEADERS,
body: JSON.stringify({ msg: prompt }),
});
const imageResponseData = await imageRes.json();
console.log('\n=====================');
console.log('IMAGE GENERATION MESSAGE DATA');
console.log(imageResponseData);
console.log('=====================');
const completedImageData = await fetchToCompletion(
imageResponseData.messageId,
0,
);
console.log('\n=====================');
console.log('COMPLETED IMAGE DATA');
console.log(completedImageData);
console.log('=====================');
/**
* INVOKE A VARIATION
*/
const variationRes = await fetch(`${BASE_URL}/button`, {
method: 'POST',
headers: AUTH_HEADERS,
body: JSON.stringify({
button: 'V1',
buttonMessageId: completedImageData.response.buttonMessageId,
}),
});
const variationResponseData = await variationRes.json();
console.log('\n=====================');
console.log('IMAGE VARIATION MESSAGE DATA');
console.log(variationResponseData);
console.log('=====================');
const completedVariationData = await fetchToCompletion(
variationResponseData.messageId,
0,
);
console.log('\n=====================');
console.log('COMPLETED VARIATION DATA');
console.log(completedVariationData);
console.log('=====================');
}
main();
Logs
The generated logs will look as follows.0
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
=====================
IMAGE GENERATION MESSAGE DATA
{
success: true,
messageId: 'ptbpfClHNs9OgbqvN0H6',
createdAt: '2023-09-02T21:25:28.675Z'
}
=====================
---------------------
Progress: 31%
Progress Image Url: https://cdn.discordapp.com/attachments/slug/slug2/hash_grid_0.webp
---------------------
---------------------
Progress: 62%
Progress Image Url: https://cdn.discordapp.com/attachments/slug/slug2/hash_grid_0.webp
---------------------
---------------------
Progress: 93%
Progress Image Url: https://cdn.discordapp.com/attachments/slug/slug2/hash_grid_0.webp
---------------------
=====================
COMPLETED IMAGE DATA
{
progress: 100,
response: {
accountId: '-------',
createdAt: '2023-09-02T21:26:03.879Z',
originatingMessageId: 'ptbpfClHNs9OgbqvN0H6',
buttons: [
'U1', 'U2', 'U3',
'U4', '🔄', 'V1',
'V2', 'V3', 'V4'
],
imageUrl: 'https://cdn.discordapp.com/attachments/slug/slug2/prompt.png',
imageUrls: [
'https://cdn.(Redacted Name).com/hash/0_0.png',
'https://cdn.(Redacted Name).com/hash/0_1.png',
'https://cdn.(Redacted Name).com/hash/0_2.png',
'https://cdn.(Redacted Name).com/hash/0_3.png'
],
description: '',
type: 'imagine',
content: 'A Rhinoceros in the Amazon with sun shining through, photorealistic, 4k',
responseAt: '2023-09-02T21:26:04.205Z',
buttonMessageId: 'pjOBnA96E5nPM5zuLnDI'
}
}
=====================
=====================
IMAGE VARIATION MESSAGE DATA
{
success: true,
messageId: 'A8ZIWcQVOrpPxEBQMbbY',
createdAt: '2023-09-02T21:26:06.283Z'
}
=====================
---------------------
Progress: 31%
Progress Image Url: https://cdn.discordapp.com/attachments/slug/slug2/hash_grid_0.webp
---------------------
---------------------
Progress: 46%
Progress Image Url: https://cdn.discordapp.com/attachments/slug/slug2/hash_grid_0.webp
---------------------
---------------------
Progress: 62%
Progress Image Url: https://cdn.discordapp.com/attachments/slug/slug2/hash_grid_0.webp
---------------------
---------------------
Progress: 78%
Progress Image Url: https://cdn.discordapp.com/attachments/slug/slug2/hash_grid_0.webp
---------------------
---------------------
Progress: 93%
Progress Image Url: https://cdn.discordapp.com/attachments/slug/slug2/hash_grid_0.webp
---------------------
=====================
COMPLETED VARIATION DATA
{
progress: 100,
response: {
accountId: 'accountId',
createdAt: '2023-09-02T21:26:40.866Z',
originatingMessageId: 'A8ZIWcQVOrpPxEBQMbbY',
ref: '',
buttons: [
'U1', 'U2', 'U3',
'U4', '🔄', 'V1',
'V2', 'V3', 'V4'
],
imageUrl: 'https://cdn.discordapp.com/attachments/slug/hash/prompt.png',
imageUrls: [
'https://cdn.(Redacted Name).com/hash/0_0.png',
'https://cdn.(Redacted Name).com/hash/0_1.png',
'https://cdn.(Redacted Name).com/hash/0_2.png',
'https://cdn.(Redacted Name).com/hash/0_3.png'
],
responseAt: '2023-09-02T21:26:41.043Z',
description: '',
type: 'button',
content: 'A Rhinoceros in the Amazon with sun shining through, photorealistic, 4k',
buttonMessageId: 'FsP2Qow7vv0n78O6Q9Md'
}
}